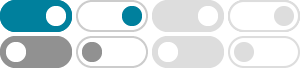
Python Program to Calculate the Radius of a Circle
You can calculate the radius of a circle using the same formula A = π r². So let’s dive into it and find out how you can calculate the radius of a circle in Python. Simple Code
how to make a radius of the circle using python - Stack Overflow
Apr 22, 2020 · pi = 3.14 c = float(input("input the circumference of the circle: ")) r = float(input("input the radius of the circle: ")) print("the diameter of the circle with circumference", c, "is:", str(2 * pi * r)) print("the area of the circle with radius", r, "is:", str(pi * r ** 2))
python - Calculate circle given 3 points (code explanation)
Oct 25, 2018 · radius = ((cx - b[0])**2 + (cy - b[1])**2)**.5. return radius. Based on Stackoverflow and Dr.Math. The code works perfectly, but I don't understand how the code fits to the explanation given at Dr.Math. Can anyone help me to understand why the code is working and what substeps are implemented in the variables?
Python - Writing a function to calculate and return the area of a circle
Jan 10, 2014 · The radius of the circle should be given as an argument to the function and the equation to calculate the area is PI*r2 area = PI*r2 def SetArea (myradius, myarea): PI = 3.14159 myarea = PI*myradius *2 return myarea
Python Programs to Calculate Area and Perimeter of Circle
5 days ago · Below are the steps to calculate the Area and Perimeter of Circle in Python: Import the math Module. Python has a built-in math module that provides the constant π (pi). To use π, import the module: import math. Define the Radius. Assign a value to the radius of the circle. Apply the Formula to calculate the Area. Use the formula A = πr².
Area of a Circle in Python - Python Guides
Aug 9, 2024 · To calculate the area of a circle in Python using basic arithmetic, you can use the formula (\text {Area} = \pi \times r^2). Here’s a simple example: This code snippet defines the radius and Pi, then calculates and prints the area of the circle. In general, the formula to calculate the area of a circle is: Where:
Python Circle Radius Calculation - CodePal
Learn how to calculate the radius of a circle in Python using the init and return methods.
Python Program to find Diameter Circumference and Area Of a Circle
Write Python Program to find Diameter Circumference and Area Of a Circle with a practical example. The mathematical formulas are: This Python program allows users to enter the radius of a circle. By using the radius value, this Python code finds the …
How to calculate the radius of a circle in Python?
Write a program to compute the radius and the central coordinate (x, y) of a circle which is constructed by three given points on the plane surface. x1, y1, x2, y2, x3, y3 separated by a single space.
Python program to enter the radius of a circle and find its …
r = radius of the circle. D = diameter of the circle. C = circumference of the circle. A = area of the circle. There are two ways to implement these formulae: By using the default value of PI = 3.14; Second, by using math.pi constant; 1. By using the default value of PI = 3.14
- Some results have been removed