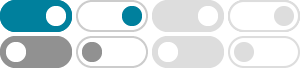
Java Program to Split an Array from Specified Position
Nov 26, 2024 · The simplest way to split an array in Java from a specified position is by using the in-built Arrays.copyOfRange () method.
How to Split an Array in Java - HowToDoInJava
Feb 22, 2023 · In this tutorial, we learned to split an array in Java for different usecases. We learned to use the Arrays.copyOfRange() API to split the arrays into any number of parts. There are other ways for array splitting as well, such that converting the array to List and the split the list.
Split an array into two parts in Java - Techie Delight
Mar 29, 2024 · This post will discuss how to split an array into two parts in Java. If the array contains an odd number of items, the extra item should be part of the first array. 1. Naive solution. A naive solution is to create two new arrays and assign elements from the first half of the source array to the first array and elements from the second half of ...
Java - Join and Split Arrays and Collections - Baeldung
Apr 4, 2025 · In this tutorial, leveraging the simple String.split function and the powerful Java 8 Stream, we illustrated how to join and split Arrays and Collections.
Split array into two parts without for loop in java
Apr 20, 2011 · Splits an array in multiple arrays with a fixed maximum size. int x = array.length / max; int r = (array.length % max); // remainder. int lower = 0; int upper = 0; List<T[]> list = new ArrayList<T[]>(); int i=0; for(i=0; i<x; i++){ upper += max; list.add(Arrays.copyOfRange(array, lower, upper)); lower = upper; if(r > 0){
Java String split() Method - W3Schools
The split() method splits a string into an array of substrings using a regular expression as the separator. If a limit is specified, the returned array will not be longer than the limit. The last element of the array will contain the remainder of the string, which may still have separators in it if the limit was reached.
java - splitting arrays - Stack Overflow
Mar 30, 2009 · If you need to move groups of items into separate arrays, for example items 0 to 10 to array 1, 11 to 20 to array 2 etc., I would create four arrays and use System.arraycopy() static method: System.arraycopy(sourceArray, sourceStart, destArray, destStart, length);
java - divide array into smaller parts - Stack Overflow
Aug 4, 2010 · public static List<byte[]> divideArray(byte[] source, int chunksize) { List<byte[]> result = new ArrayList<byte[]>(); int start = 0; while (start < source.length) { int end = Math.min(source.length, start + chunksize); result.add(Arrays.copyOfRange(source, start, end)); start += chunksize; } return result; }
How to Split an Array into Two Equal Parts in Java Without Using …
Learn how to split a large array into two equal parts in Java without for loops, explore performance implications and alternative methods.
Java: how can I split an ArrayList in multiple small ArrayLists?
You can use subList(int fromIndex, int toIndex) to get a view of a portion of the original list. From the API: Returns a view of the portion of this list between the specified fromIndex, inclusive, and toIndex, exclusive. (If fromIndex and toIndex are equal, the returned list is empty.)