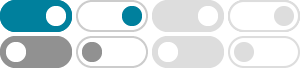
Python Program to Multiply Two Matrices - GeeksforGeeks
Sep 27, 2024 · Given two matrices, we will have to create a program to multiply two matrices in Python. Example: Python Matrix Multiplication of Two-Dimension. This Python program multiplies two matrices using nested loops. It initializes two matrices A and B, along with a …
Strassen algorithm in Python - GeeksforGeeks
Jun 1, 2024 · Strassen's algorithm is an efficient method for matrix multiplication. It reduces the number of arithmetic operations required for multiplying two matrices by decomposing them into smaller submatrices and performing recursive multiplication.
3 Ways to Multiply Matrices in Python - Geekflare
Dec 28, 2024 · In this tutorial, you’ll learn how to multiply two matrices in Python. You’ll start by learning the condition for valid matrix multiplication and write a custom Python function to multiply matrices. Next, you will see how you can achieve the same result using nested list comprehensions.
Matrix Multiplication in Python: A Comprehensive Guide
Jan 26, 2025 · In Python, there are several ways to perform matrix multiplication, each with its own advantages and use cases. This blog post will explore the concepts, usage methods, common practices, and best practices for matrix multiplication in Python.
Python Program to Multiply Two Matrices
Here are a couple of ways to implement matrix multiplication in Python. [4 ,5,6], [7 ,8,9]] # 3x4 matrix . [6,7,3,0], [4,5,9,1]] # result is 3x4 . [0,0,0,0], [0,0,0,0]] # iterate through rows of X for i in range(len(X)): # iterate through columns of Y for j in range(len(Y[0])): # iterate through rows of Y for k in range(len(Y)):
Matrix Multiplication in Python (with and without Numpy)
Matrix multiplication, also known as matrix dot product, is a binary operation that takes a pair of matrices and produces another matrix. In Python, this operation can be performed using the NumPy library, which provides a function called dot for matrix multiplication.
Matrix multiplication in Python with user input
In this post, you will learn the python program to multiply two matrices by taking input from the user but before writing a program let’s understand the rule for matrix multiplication and the algorithm to implement it.
The Strassen’s Algorithm with a Python Example - Medium
Sep 3, 2024 · Strassen’s Algorithm breaks down the original matrices into smaller pieces and strategically combines them to minimize the number of multiplications needed. For a 2x2 matrix, instead of...
Matrix Multiplication in NumPy - GeeksforGeeks
Sep 2, 2020 · Let us see how to compute matrix multiplication with NumPy. We will be using the numpy.dot () method to find the product of 2 matrices. In Python numpy.dot () method is used to calculate the dot product between two arrays. Example 1 : Matrix multiplication of 2 square matrices. Output : [26 31]]
Matrix Multiplication in Python without libraries - Medium
Dec 24, 2023 · Implementing matrix multiplication without external libraries can be a useful exercise that allows you to understand the fundamental principles behind matrix operations and algorithms.