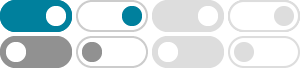
how to search a number using binary search algorithm (without ...
Jun 16, 2016 · Below is my complete code for binary search written in python3 version. I was able to:-i) create a list, ii) sorted the list using bubble sort algorithm, iii)wrote code snippet for …
Binary Search (Recursive and Iterative) - Python - GeeksforGeeks
Feb 21, 2025 · The binary_search_bisect() function is defined which takes an array arr and the element to search x as inputs. The function calls the bisect_left() function of the bisect module …
5 Best Ways to Implement Binary Search in Python Without …
Mar 7, 2024 · Method 1: Iterative Binary Search Using While Loop. Efficient and straightforward. Quickly narrows the search space. Method 2: Iterative Binary Search Using For Loop. …
Implement Binary Search Without Recursion in Python
Dec 9, 2024 · To implement binary search without recursion, the program takes a sorted list and a key as input. It uses the Binary search method, which is an efficient way to find a specific item …
binary search implementation with python - Stack Overflow
Jul 15, 2015 · def Binary_search(num,desired_value,left,right): while left <= right: mid = (left + right)//2 if desired_value == num[mid]: return mid elif desired_value > num[mid]: left = mid + 1 …
Binary search algorithm in python - Stack Overflow
Feb 29, 2012 · def binary_search(number): numbers_list = range(20, 100) i = 0 j = len(numbers_list) while i < j: middle = int((i + j) / 2) if number > numbers_list[middle]: i = middle …
Python Program For Binary Search (With Code) - Python Mania
Binary search is a search algorithm that finds the position of a target value within a sorted collection of elements. The algorithm compares the target value with the middle element of the …
Binary Search in Python - PrepInsta
This Python binary search program takes user input for a target number and a sorted list of numbers. It then efficiently finds the target value within the list by iteratively adjusting the …
Python Program to Perform Binary Search without Recursion
This is a Python program to implement binary search without recursion. The program takes a list and key as input and finds the index of the key in the list using binary search. 1. Create a …
Binary Search Functions and Data Structures in Python
May 4, 2023 · Binary search mostly works with integer data types. The basic functionality of binary search includes searching a sorted array for a given number. The brute force approach …