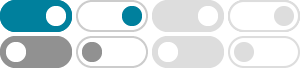
Reverse a String in Java - GeeksforGeeks
Mar 27, 2025 · We can use character array to reverse a string. Follow Steps mentioned below: First, convert String to character array by using the built-in Java String class method toCharArray(). Then, scan the string from end to start, and print the character one by one.
Reverse a string in Java - Stack Overflow
Sep 10, 2017 · public static String reverse(String input){ char[] in = input.toCharArray(); int begin=0; int end=in.length-1; char temp; while(end>begin){ temp = in[begin]; in[begin]=in[end]; in[end] = temp; end--; begin++; } return new String(in); }
Reverse a String in Java in 10 different ways | Techie Delight
Apr 1, 2024 · This post covers 10 different ways to reverse a string in java by using StringBuilder, StringBuffer, Stack data structure, Java Collections framework reverse() method, character array, byte array, + (string concatenation) operator, Unicode right-to-left override (RLO) character, recursion, and substring() function.
How to Reverse a String in Java: 9 Ways with Examples [Easy]
This tutorial covers 9 methods for how to reverse a string in Java, including methods using built-in reverse functions, recursion, and a third-party library.
How To Reverse A String In Java – Learn 5 Easy Methods
May 30, 2021 · To use the reverse( ) function of the StringBuilder class, first can convert the given String input to StringBuilder object, then reverse it by using a reverse( ) function, and finally, convert that reversed StringBuilder object to String.
How to Reverse a String in Java:13 Best Methods - Simplilearn
Apr 14, 2025 · You can use Collections.reverse() to reverse a Java string. Use these steps: Create an empty ArrayList of characters, then initialize it with the characters of the given string with String.toCharArray().
How to Reverse a String in Java: A Step-by-Step Guide
There are three prominent methods to reverse a string in Java: 1. Using a Loop: Iterates through the string from end to start. 2. Using StringBuilder: Utilizes the built-in reverse() function of the StringBuilder class for better performance. 3. Using Recursion: A more advanced technique that calls the function itself to reverse the string.
How to Reverse a String in Java - Baeldung
Jan 8, 2024 · In this quick tutorial, we’re going to see how we can reverse a String in Java. We’ll start to do this processing using plain Java solutions. Next, we’ll have a look at the options that third-party libraries like Apache Commons provide. Furthermore, we’ll demonstrate how to reverse the order of words in a sentence. 2. A Traditional for Loop.
Reverse String in Java | Using Various Methods with Examples
We can achieve the string reverse in Java using StringBuilder. We can create an object of the StringBuilder and use the string.reverse () function for the string reverse. Since this is a string function, you need not import any additional library.
Best Way to Reverse a String in Java - Java Guides
There are several methods to reverse a string, each with its own advantages and use cases. This blog post will explore the best ways to reverse a string in Java, including using built-in methods, looping constructs, and external libraries. 1. Using StringBuilder or StringBuffer.
- Some results have been removed