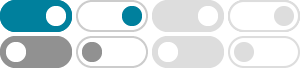
Java Inheritance (Subclass and Superclass) - W3Schools
In Java, it is possible to inherit attributes and methods from one class to another. We group the "inheritance concept" into two categories: To inherit from a class, use the extends keyword. In the example below, the Car class (subclass) inherits the attributes and methods from the Vehicle class (superclass):
Java Inheritance (With Examples) - Programiz
In Java, inheritance is an is-a relationship. That is, we use inheritance only if there exists an is-a relationship between two classes. For example, Car is a Vehicle; Orange is a Fruit; Surgeon is a Doctor; Dog is an Animal; Here, Car can inherit from Vehicle, Orange can inherit from Fruit, and so …
inheritance - Creating a Java Car class - Stack Overflow
Jul 23, 2014 · The car class is for a Car Hire Company to store the information about the car like the make, model and registration number, so that using the driver class I can use to input new vehicles, check if a vehicle is on hire and unavailable and name of hirer if it is hired. My car class with methods: //Constructor,
Java Program: Method Overriding with Vehicle and Car
Feb 19, 2025 · Write a Java program where the "Car" subclass includes a method to check fuel level and alert when low. Write a Java program where the "Vehicle" class has a method to check engine status, and subclasses override it.
Inheritance in Java Example - DigitalOcean
Aug 3, 2022 · Inheritance in Java is the method to create a hierarchy between classes by inheriting from other classes. Java Inheritance is transitive - so if Sedan extends Car and Car extends Vehicle, then Sedan is also inherited from the Vehicle class. The Vehicle becomes the superclass of both Car and Sedan.
Inheritance in Java with Example - Java Guides
7. Real-World Examples of Inheritance Example 1: Vehicle Hierarchy. Consider a vehicle hierarchy where Vehicle is the base class. Car and Bike can be derived classes that inherit properties and methods from Vehicle. Example 2: Employee Hierarchy. In an employee management system, Employee can be the base class.
Java Inheritance Tutorial: Explained with examples - Educative
To declare inheritance in Java, we simply add extends [superclass] after the subclass’s identifier. Here’s an example of a class Car that inherits from base class Vehicle using private strings and getter/setter methods to achieve encapsulation.
Java Inheritance Programming - Vehicle class hierarchy
Feb 19, 2025 · Write a Java program to create a vehicle class hierarchy. The base class should be Vehicle, with subclasses Truck, Car and Motorcycle. Each subclass should have properties such as make, model, year, and fuel type. Implement methods for calculating fuel efficiency, distance traveled, and maximum speed. Sample Solution: Java Code:
Inheritance in Java with Examples - Software Test Academy
Sep 16, 2021 · Inheritance in Java with Examples. So, let’s implement our Car class and let say that all cars can speed up and consume energy and they have a name. Below, I also used @Getter and @Setter Lombok annotation, rather than writing these boring get and set methods. I also suggest using Lombok Library in your Java projects.
Java Inheritance Explained - Master OOP with Real-World Examples | Java …
Mar 16, 2025 · Real-World Example: Vehicles 🚗. A Car and a Bike share common attributes like speed, color, fuel type. Instead of redefining them, we create a Vehicle class and let Car and Bike inherit...