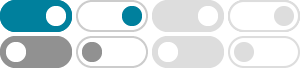
How to convert a Python dict to a Class object - Stack Overflow
Dec 9, 2019 · I am looking for a simple way to directly convert a python dict to a custom object as follows, while not breaking intellisense. The result should not be read-only, it should behave just like a new object.
Python dictionary from an object's fields - Stack Overflow
Sep 15, 2008 · To build a dictionary from an arbitrary object, it's sufficient to use __dict__. Usually, you'll declare your methods at class level and your attributes at instance level, so __dict__ should be fine.
How to convert a nested Python dict to object? - Stack Overflow
In 2021, use pydantic BaseModel - will convert nested dicts and nested json objects to python objects and vice versa: https://pydantic-docs.helpmanual.io/usage/models/ >>> class Foo(BaseModel): ... count: int ... size: float = None ... >>> >>> class Bar(BaseModel): ... apple = 'x' ... banana = 'y' ... >>> >>> class Spam(BaseModel): ... foo: Foo ...
5 Best Ways to Convert Python Dict to Object – Be on the
Feb 21, 2024 · This is a straightforward way to convert a dictionary to an object, promoting readability and ease of use for those familiar with basic Python classes. Here’s an example: class DictToObject: def __init__(self, dictionary): for key, value in dictionary.items(): setattr(self, key, value) dict_example = {'name': 'Alice', 'age': 30} obj ...
Python Dictionaries - W3Schools
From Python's perspective, dictionaries are defined as objects with the data type 'dict': Print the data type of a dictionary: It is also possible to use the dict () constructor to make a dictionary. Using the dict () method to make a dictionary: There are four collection data types in the Python programming language:
5 Best Ways to Convert a Python Dict to an Object with Attributes
Feb 21, 2024 · The problem is transforming a Python dictionary, e.g., {'name': 'Alice', 'age': 30}, into an object so that we can access its attributes using dot notation like object.name or object.age. This article outlines five different methods for achieving this transformation.
How to Create a Dictionary in Python - GeeksforGeeks
Feb 8, 2025 · For example, given two lists, keys = [‘a’, ‘b’, ‘c’] and values = [1, 2, 3], the goal is to construct a dictionary like {‘a’: 1, ‘b’: 2, ‘c’: 3}, mapping each key to its corresponding value. dict () constructor provides a simple and direct way to create dictionaries using keyword arguments.
Python Dictionary as Object - Joel McCune
Mar 8, 2021 · Python dictionaries do not help much with this, but Python Objects do. This logically begs the question, how to create the nested object heirarchy so I can access the same value using dot syntax. my_dict.nested_value.n1
Python Dictionary: How To Create And Use, With Examples
Oct 22, 2024 · Creating a Python Dictionary. Let’s look at how we can create and use a Python dictionary in the Python REPL: >>> phone_numbers = { 'Jack': '070-02222748', 'Pete': '010-2488634' } >>> my_empty_dict = { } >>> phone_numbers['Jack'] '070-02222748' A dictionary is created by using curly braces.
dict() Constructor in Python - GeeksforGeeks
Nov 18, 2024 · In Python, the dict () constructor is used to create a dictionary object. A dictionary is a built-in data type that stores data in key-value pairs, similar to a real-life dictionary where each word (the key) has a meaning (the value).
- Some results have been removed