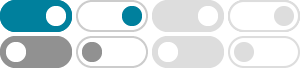
python - Cannot concatenate 'str' and 'float' objects? - Stack Overflow
With Python3.6+, you can use f-strings to format print statements. print(f"You need {3.14*height*radius**2:8.2f} gallons of water to fill this pool.") See similar questions with these …
Join float list into space-separated string in Python
print " ".join(map(str, a)) If you want more control over the conversion to string (e.g. control how many digits to print), you can use print "".join(format(x, "10.3f") for x in a)
How to Concatenate String and Float in Python? - Python Guides
Aug 12, 2024 · In this tutorial, I will show you how to concatenate string and float in Python using various methods with examples. To concatenate a string and a float in Python, you can use …
Join lists and individual floats to single string in Python
Mar 26, 2013 · >>> a = [[1.0, 1.2, 1.4], [2.0, 2.2], 5.0] >>> ' '.join(str(s) for x in a for s in (x if isinstance(x, list) else [x])) '1.0 1.2 1.4 2.0 2.2 5.0' This is equivalent in behavior to the …
5 Best Ways to Join a List of Floats to a String in Python
Feb 24, 2024 · The str.join() method allows to concatenate an iterable of strings, which can be created by casting each float in the list to a string using list comprehension. It’s a clean and …
Python Concatenate String and int, float Variable - Tuts Make
Nov 3, 2022 · In this python string concatenate/join with other dataTypes variable. Here you will learn how to concatenate strings and int, how to concatenate strings and float and etc. Python …
Python String Concatenation - GeeksforGeeks
Nov 5, 2024 · Use join () function to concatenate strings with a specific separator. It’s especially useful when working with a sequence of strings, like a list or tuple. If no separator is needed …
How to Concatenate List in Python - Codecademy
Mar 20, 2025 · This code uses the * operator to unpack and merge the elements of lists a and b into a new list, c.. While the * operator is concise, a for loop offers more control when custom …
Concatenate strings in Python (+ operator, join, etc.) - nkmk note
Aug 15, 2023 · Call the join() method on the string you wish to insert between the elements (e.g., 'STRING_TO_INSERT') and pass a list of strings (e.g., [LIST_OF_STRINGS]). Using an …
Python String join() Method - W3Schools
Join all items in a tuple into a string, using a hash character as separator: The join() method takes all items in an iterable and joins them into one string. A string must be specified as the …