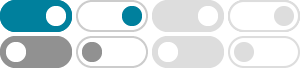
How do I declare and initialize an array in Java? - Stack Overflow
Jul 29, 2009 · There are various ways in which you can declare an array in Java: float floatArray[]; // Initialize later int[] integerArray = new int[10]; String[] array = new String[] {"a", "b"}; You can find more information in the Sun tutorial site and the JavaDoc.
Arrays in Java - GeeksforGeeks
Mar 28, 2025 · To declare an array in Java, use the following syntax: type [] arrayName; type: The data type of the array elements (e.g., int, String). arrayName: The name of the array. Note: The array is not yet initialized. 2. Create an Array. To create an array, you need to allocate memory for it using the new keyword:
How to Initialize an Array in Java? - GeeksforGeeks
Apr 14, 2025 · In this article, we will discuss different ways to declare and initialize an array in Java. Understanding how to declare an array in Java is very important. In Java, an array is declared by specifying its data type, and an identifier, and adding brackets [] …
Java Arrays - W3Schools
Java Arrays. Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value. To declare an array, define the variable type with square brackets:
Initializing Arrays in Java - Baeldung
Dec 16, 2024 · int[] array = new int[5]; Arrays.fill(array, 0, 3, -50); Here, the fill() method accepts the initialized array, the index to start the filling, the index to end the filling (exclusive), and the value itself respectively as arguments.
How to Declare an Array in Java? - GeeksforGeeks
Feb 28, 2025 · Declaring an array in Java means defining an array variable without assigning values. It simply creates a reference to an array but does not allocate memory. The actual memory allocation happens when the array is initialized. Declaration of …
Creating an array of objects in Java - Stack Overflow
There are 3 steps to create arrays in Java - Declaration – In this step, we specify the data type and the dimensions of the array that we are going to create. But remember, we don't mention the sizes of dimensions yet. They are left empty. Instantiation – In this step, we create the array, or allocate memory for the array, using the new keyword.
How to Create an Array in Java – Array Declaration Example
Mar 16, 2023 · In this article, we will provide a step-by-step guide on how to create an array in Java, including how to initialize or create an array. We will also cover some advanced topics such as multi-dimensional arrays, array copying, and array sorting.
How to initialize an array in Java? - Stack Overflow
Dec 21, 2009 · If you want to initialize an array, try using Array Initializer: Notice the difference between the two declarations. When assigning a new array to a declared variable, new must be used. Even if you correct the syntax, accessing data[10] is still incorrect (You can only access data[0] to data[9] because index of arrays in Java is 0-based).
How to Create an Array in Java – Array Declaration Example
Aug 24, 2024 · Knowing how to create, initialize, and manipulate arrays is critical for any Java programmer. In this comprehensive tutorial, we will explore arrays in Java in depth. We will cover: