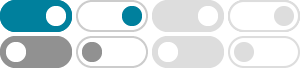
JavaScript Promises - W3Schools
Here is how to use a Promise: Promise.then () takes two arguments, a callback for success and another for failure. Both are optional, so you can add a callback for success or failure only. To demonstrate the use of promises, we will use the callback examples from the previous chapter:
Promise - JavaScript | MDN - MDN Web Docs
Apr 10, 2025 · Promises in JavaScript represent processes that are already happening, which can be chained with callback functions. If you are looking to lazily evaluate an expression, consider using a function with no arguments e.g., f = => expression to create the lazily-evaluated expression, and f() to evaluate the expression immediately.
JavaScript Promise - GeeksforGeeks
Jan 18, 2025 · JavaScript Promises make handling asynchronous operations like API calls, file loading, or time delays easier. Think of a Promise as a placeholder for a value that will be available in the future. It can be in one of three states. Pending: The task is in the initial state. Fulfilled: The task was completed successfully, and the result is available.
Using promises - JavaScript | MDN - MDN Web Docs
Apr 3, 2025 · A Promise is an object representing the eventual completion or failure of an asynchronous operation. Since most people are consumers of already-created promises, this guide will explain consumption of returned promises before explaining how to create them.
javascript - Creating a (ES6) promise without starting to resolve …
Jun 26, 2015 · Using ES6 promises, how do I create a promise without defining the logic for resolving it? Here's a basic example (some TypeScript): if (key in promises) { return promises[key]; var promise = new Promise(resolve => { // But I don't want to try resolving anything here :( }); promises[key] = promise; return promise;
How Promises Work in JavaScript – A Comprehensive Beginner's …
Jun 13, 2023 · This article is an in-depth guide to promises in JavaScript. You are going to learn why JavaScript has promises, what a promise is, and how to work with it. You are also going to learn how to use async/await—a feature derived from promises—and what a job queue is. Here are the topics we will cover: Why should you care about promises?
How to Write a JavaScript Promise - freeCodeCamp.org
Feb 5, 2019 · Promises give us a way to wait for our asynchronous code to complete, capture some values from it, and pass those values on to other parts of our program. I have an article here that dives deeper into these concepts: Thrown For a Loop: Understanding Loops and Timeouts in JavaScript. How do we use a promise?
How JavaScript Promises Work – Handbook for Beginners
Feb 13, 2024 · In this handbook, you'll learn all about JavaScript Promises and how to use them. What is a Promise? Let's begin by looking at what a Promise is. In simple terms, a Promise is an object representing an asynchronous operation. This object can tell you when the operation succeeds, or when it fails.
JavaScript Create Promise - Mastering JS
Mar 27, 2020 · In general, there are 4 ways to create a new promise in JavaScript: Using the Promise constructor; Using the static helpers Promise.resolve() and Promise.reject() Chaining with the then() function or catch() function; Call an async function; Using the Promise Constructor. The Promise constructor takes a single parameter, an executor function.
This is How To Make JS Promises [From Scratch]
Aug 2, 2021 · Let's dive in, simplify, and set up our implementation of a JavaScript Promise to always return or resolve an additional Promise from a .then() statement. To start with, we want a method that will transform the value contained by the promise and give us back a new Promise.
- Some results have been removed