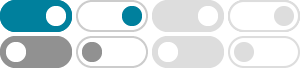
python - How do I compute derivative using Numpy ... - Stack Overflow
Mar 26, 2012 · Assuming you want to use numpy, you can numerically compute the derivative of a function at any point using the Rigorous definition: def d_fun(x): h = 1e-5 #in theory h is an infinitesimal return (fun(x+h)-fun(x))/h
A Quick Guide to Calculating Derivatives in Python - Turing
Put simply, taking a Python derivative measures how a function responds to infinitesimally small changes in its input. It provides valuable insights into the behavior, trends, and characteristics of mathematical functions. This article will look at the methods and techniques for calculating derivatives in Python.
How do you evaluate a derivative in python? - Stack Overflow
May 31, 2017 · It is a function that returns the derivative (as a Sympy expression). To evaluate it, you can use .subs to plug values into this expression: >>> fprime(x, y).evalf(subs={x: 1, y: 1}) 3.00000000000000 If you want fprime to actually be the derivative, you should assign the derivative expression directly to fprime, rather than wrapping it in a ...
Derivatives in Python using SymPy - AskPython
Nov 12, 2020 · There are certain rules we can use to calculate the derivative of differentiable functions. Some of the most encountered rules are: Let’s dive into how can we actually use sympy to calculate derivatives as implied by the general differentiation rules. 1. Power Rule. In general : f' (x n) = nx (n-1) Example, Function we have : f (x) = x⁵.
How to calculate and plot the derivative of a function using …
Aug 6, 2024 · In this article, we will learn to calculate the derivative of a function and then plot that derivative of a function using the Matplotlib library in Python. Here, we are going to determine the derivative of a function using Python.
Mastering Derivatives in Python: Concepts, Usage, and Best …
1 day ago · In the realm of mathematics and data analysis, derivatives play a crucial role. They help us understand how a function changes with respect to its input variable. In Python, we have various tools and libraries to calculate derivatives, which are essential for tasks like optimization, numerical analysis, and machine learning. This blog post …
Calculate Derivatives using Python - idroot
In Python, we can approach derivative calculations in two main ways: symbolic and numerical differentiation. Symbolic differentiation provides exact solutions, while numerical methods offer approximations useful for complex functions or when working with discrete data points. Python offers several libraries that excel at calculating derivatives.
Calculate Derivative Functions in Python - Joshua Bowen's Notes
Jan 1, 2022 · To compute derivates, use the diff function. The first parameter of the diff function should be the function you want to take the derivative of. The second parameter should be the variable you are taking the derivative with respect to. The output for the f …
How to Calculate Derivative in Python - Delft Stack
Feb 26, 2025 · Learn how to calculate derivatives in Python using the SymPy library. This article provides step-by-step instructions and code examples for differentiating simple and complex functions, including polynomials and trigonometric functions.
Taking Derivatives in Python | Towards Data Science
Oct 7, 2019 · Now use the power rule to calculate the derivative. It’s pretty straightforward: Now let’s take a look at how to calculate it in Python. The first thing is to import the library, and then to declare a variable that you will use as a letter in your functions. Here’s how to do it for a single variable function: