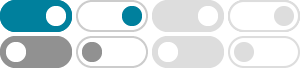
Generate random number in range excluding some numbers
Mar 24, 2017 · Is there a simple way in Python to generate a random number in a range excluding some subset of numbers in that range? For example, I know that you can generate a random number between 0 and 9 with: from random import randint randint(0,9) What if I have a list, e.g. exclude=[2,5,7], that I don't want to be returned?
Choosing random integers except for a particular number for python?
Jul 28, 2013 · Use random.choice on a list, but first remove that particular number from the list: Or define a function: return list(range(start, n)) + list(range(n+1, end)) ... Update: This returns all numbers other than a particular number from the list: others = list(range(0, player)) + list(range(player+1, 5)) print player,'-->', others. ...
Can Python generate a random number that excludes a set of numbers …
May 19, 2012 · A possible solution would be to just shift the random numbers out of that range. E.g. def NormalWORange(a, b, sigma): r = random.normalvariate(a,sigma) if r < a: return r-b else: return r+b
How do I exclude several numbers from random randint?
return random_exclude(range_start, range_end, excludes) This function will call itself if the result of random.randint is in excludes list. Otherwise it returns the random number. You could do the same with a while loop. r = random.randint(range_start, range_end) while r in excludes: r = random.randint(range_start, range_end) return r.
Python | Generate random number except K in list
May 18, 2023 · Method #1 : Using choice () + list comprehension. The combination of the above functions can be used to perform this task. In this, we first filter out the numbers except K using list comprehension and then feed that list to choice () for random number generation. Time Complexity: O (n) where n is the number of elements in the list “test_list”.
Python: Generate a number in a specified range except some specific ...
3 days ago · # Define a function 'generate_random' that generates a random number within a specified range, excluding certain numbers def generate_random(start_range, end_range, nums): # Generate a random number within the specified range, excluding numbers in the 'nums' list .
Mastering Randomness in Python: Generating Unique and Excluded Numbers
We can create a set object with the numbers in a range, exclude the numbers we want to exclude, and then use the random.choice() method to select a random number from the set. Example: Suppose we want to generate a random number between 1 and 10, excluding the numbers 3 …
How do you create a random range, but exclude a specific number?
Apr 24, 2015 · How do you create a random range, but exclude a specific number? Using random.choice: import random allowed_values = list(range(-5, 5+1)) allowed_values.remove(0) # can be anything in {-5, ..., 5} \ {0}: random_value = random.choice(allowed_values)
Generate random numbers except a particular number in a Python …
Hello everyone, in this tutorial, we will learn to generate random numbers except a particular number in a Python list. We are going to use random.choice() method with the list comprehension technique to get the desired result.
python | How to exclude certain numbers with random.randint?
import random elementos = [n for n in range(0, 101) if n not in [50, 99, 80]] x = elementos[random.randint(0, len(elementos))] It may eventually be more optimal if the number of excluded values is significant.
- Some results have been removed