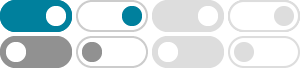
B Tree in Python - GeeksforGeeks
Apr 17, 2024 · Let us see how we can define a class for the B tree in Python. Let us see the individual parts of code used for the implementation of the B tree. Create a node with the name 'BTreeNode' to create a default node with a list of keys and a list of children nodes.
How can I implement a tree in Python? - Stack Overflow
Mar 1, 2010 · bigtree is a Python tree implementation that integrates with Python lists, dictionaries, and pandas DataFrame. It is pythonic, making it easy to learn and extendable to many types of workflows. There are various components to bigtree, namely
python - How to implement a binary tree? - Stack Overflow
Apr 8, 2010 · Here is my simple recursive implementation of binary search tree. def __init__(self, val): self.l = None. self.r = None. self.v = val. def __init__(self): self.root = None. def get_root(self): return self.root. def add(self, val): if not self.root: self.root = Node(val) else: self._add(val, self.root) def _add(self, val, node): if val < node.v:
Introduction to B-Trees - Python Examples
A B-Tree is a self-balancing tree data structure that maintains sorted data and allows for efficient insertion, deletion, and search operations. B-Trees are widely used in databases and file systems to store large amounts of data that cannot fit entirely into memory.
B Tree in Python using OOP Concepts - OpenGenus IQ
In this article at OpenGenus, we will explore the implementation of a B-tree in Python using the principles of Object-Oriented Programming (OOP). By utilizing classes and objects, we'll create a B-tree structure that supports efficient insertion, searching, and display operations.
A simple B-Tree in Python that supports insert, search and print.
"""A BTree implementation with search and insert functions. Capable of any order t.""" class Node(object): """A simple B-Tree Node.""" def __init__(self, t): self.keys = [] self.children = [] self.leaf = True # t is the order of the parent B-Tree. Nodes need this value to define max size and splitting. self._t = t: def split(self, parent, payload):
B-tree Implementation in Python – Learn Programming
The implementation of a B-tree is structured around two main classes: BTreeNode and BTree. 1. BTreeNode Class. The BTreeNode class represents a node in the B-tree. Each node contains: keys: A list that stores the keys in the node, kept in sorted order. children: A list of pointers to child nodes. This list is empty for leaf nodes.
B Tree in Data Structure: Properties, Examples, Full Guide
Mar 8, 2025 · Insertion in B-Tree involves adding a new key into the appropriate position while maintaining the tree's properties. If a node becomes full, it is split into two nodes, and the middle key is promoted to the parent node. Search for the correct leaf node to insert the new key. Insert the key into the leaf node in sorted order.
How to Implement a B-Tree Data Structure (2023 Version)
Oct 19, 2022 · This tutorial discussed B-trees and the application of B-trees for storing a large amount of data on disks. We also discussed four primary operations in B-trees: traversing, searching, inserting, and deleting.
Understanding Binary Tree: Guide to Binary Tree Implementation …
Oct 18, 2024 · In a Binary Search Tree (BST), the search starts at the root and moves left if the value is smaller, right if larger, continuing recursively until found or null. In-order (Left → Node →...
- Some results have been removed