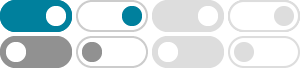
Extend range in Python - Stack Overflow
Jan 16, 2014 · range() in Python 3 returns a dedicated immutable sequence object. You'll have to turn it into a list to extend it: Note that I used list.append(), not list.extend() (which expects a sequence of values, not one integer). However, you probably want …
How can I increase the size of, and pad, a python list?
Mar 14, 2013 · You can use itertools.chain() or chain.from_iterable() to flatten a list of lists (y in your case) : In [23]: lis=[1,2,3,4] In [24]: from itertools import chain In [31]: y = list(chain(*([n]*4 for n in lis))) In [32]: y Out[32]: [1, 1, 1, 1, 2, 2, 2, 2, 3, 3, 3, 3, 4, 4, 4, 4]
Extending a list in Python - GeeksforGeeks
Dec 17, 2024 · extend() method is the most efficient way to add multiple elements to a list. It takes an iterable (like a list, tuple, or set) and appends each of its elements to the existing list. Explanation: The extend() method directly modifies the original list by adding all elements from n …
Basic python: how to increase value of item in list
Basically I want to iterate through the items in an empty list and increase each one according to some criteria. This is an example of what I'm trying to do: list1 = [] for i in range(5): list1[i] = list1[i] + 2*i
Python Range List: A Comprehensive Guide - CodeRivers
Apr 5, 2025 · Python's range object and the process of creating lists from ranges are powerful and versatile features. Understanding the fundamental concepts, usage methods, common practices, and best practices related to them can greatly improve your Python programming.
Python – List of N size increasing lists | GeeksforGeeks
Apr 5, 2023 · Define a recursive function that takes in three arguments: N (the number of lists to generate), M (the maximum element in each list), and start (the starting element for the first list). The function should return a list of N size-increasing lists.
Python range() Function: How-To Tutorial With Examples
Jun 27, 2023 · Sometimes you want to create a list from a range. You could do this with a for-loop. Or, more Pythonic and quicker: by using a Python list comprehension. However, the best way to do this is using the list() function, which can convert any iterable object to a list, including ranges: my_list = list(range(0, 3)) print(my_list) # [0, 1, 2]
Creating lists of increasing length python - Stack Overflow
Sep 1, 2017 · super_list.append(list(range(i))) # .append(range(i)) suffices for python2. You may want to tweak the ranges, depending on your desired outcome. 10 iterations of that will give you ten lists, like so: [0], [0, 1], [0, 1, 2], [0, 1, 2, 3], [0, 1, 2, 3, 4], [0, 1, 2, 3, 4, 5], [0, 1, 2, 3, 4, 5, 6], [0, 1, 2, 3, 4, 5, 6, 7],
Extending a List in Python - Online Tutorials Library
Learn how to extend a list in Python with practical examples and explanations. Discover various methods to add elements efficiently.
Python | Increase list size by padding each element by N
Apr 17, 2023 · Given a list and an integer N, write a Python program to increase the size of the list by padding each element by N. Examples: Input : lst = [1, 2, 3] N = 3 Output : [1, 1, 1, 2, 2, 2, 3, 3, 3] Input : lst = ['cats', 'dogs'] N = 2 Output : ['cats', 'cats', 'dogs', 'dogs']
- Some results have been removed