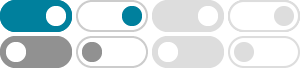
Reversing user input array in Java - Stack Overflow
Jul 15, 2013 · If you want to reverse the order of elements, that's REVERSE ORDER not SHIFT. "Shift" correctly would shift them all one to the right or left, either dropping the end element or or (possibly) rotating it round to the other end.
Reverse an array in Java - GeeksforGeeks
Nov 25, 2024 · The simplest way to reverse an array in Java is by using a loop to swap elements or by using the helper methods from the Collections and Arrays classes. Example: Let’s take an example to reverse an array using a basic loop approach.
How do I reverse an int array in Java? - Stack Overflow
Jan 26, 2010 · java.util.Collections.reverse() can reverse java.util.Lists and java.util.Arrays.asList() returns a list that wraps the the specific array you pass to it, therefore yourArray is reversed after the invocation of Collections.reverse().
java - How to reverse array input - Stack Overflow
Oct 17, 2021 · The original array gets replaced by the reversed array. Implementation of the reverse function is given below: static void reverse(int array[], int sizeOfArray) { int i, k, t; for (i = 0; i < sizeOfArray / 2; i++) { t = array[i]; array[i] = array[n - i - 1]; array[n - i - 1] = t; }
Array Reverse – Complete Tutorial - GeeksforGeeks
Sep 25, 2024 · The idea is to use recursion and define a recursive function that takes a range of array elements as input and reverses it. Inside the recursive function, Swap the first and last element. Recursively call the function with the remaining subarray.
5 Best Ways to Reverse an Array in Java - Codingface
May 20, 2022 · We can reverse the order of an Array in Java by following methods: 1. Reverse an Array by using a simple for loop through iteration. 2. Reverse an Array by using the in-place method. 3. Reverse an Array by using ArrayList and Collections.reverse( ) method 4. Reverse an Array by using StringBuilder.append( ) method 5.
Reverse an Array in Java - Tpoint Tech
In this tutorial, we will discuss how one can reverse an array in Java. In the input, an integer array is given, and the task is to reverse the input array.
Reverse An Array In Java - 3 Methods With Examples - Software …
Apr 1, 2025 · Q #1) How do you Reverse an Array in Java? Answer: There are three methods to reverse an array in Java. Using a for loop to traverse the array and copy the elements in another array in reverse order. Using in-place reversal in which the elements are swapped to place them in reverse order.
Reverse an Array in Java - 4 Methods with Code
Nov 11, 2022 · Reverse an Array by 1. Reverse Print, 2. Using Auxiliary Array, 3. Without Auxiliary Array, 4. Reverse Array Using Collections.reverse() Method
Java program to reverse array using for loop - oodlescoop
Dec 18, 2021 · This Java program demonstrates how to reverse the elements of an array manually using a for loop. The java.util.Scanner class is imported to handle user input. The program prompts the user to enter the size of the array. The input size is read using a Scanner object and stored in the variable size.