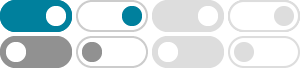
python - Understanding the map function - Stack Overflow
Jun 11, 2012 · In Python 2 map(), will iterate (go through the elements of the lists) according to the longest list, and pass None to the function for the shorter lists, so your function should look for None and handle them, otherwise you will get errors. In Python 3 map() will stop after finishing with the shortest
python - When should I use a Map instead of a For Loop ... - Stack …
EDIT: I didn't realize that map equals itertools.imap after python 3.0. So the conclusion here may not be correct. I'll re-run the test on python 2.6 tomorrow and post result. If doSomething is very "tiny", map can be a lot faster than for loop or a list-comprehension:
python map function (+ lambda) involving conditionals (if)
Note that as you don't need the result of filtered list and you just want to pass it to map it's more efficient that use ifilter because it returns a generator and you can save much memory for long lists ;) (its all in python 2 and in python 3 filter returns generator)
python - Why use the map () function? - Stack Overflow
Jun 9, 2014 · You will notice that Python has some other functional programming functions such as reduce, filter, zip etc. map is part of this class of functions where although each implements a very simple function, when you combine these into a …
python - How to do multiple arguments to map function where …
we want to apply map function for an array. map(add, [1, 2, 3], 2) The semantics are I want to add 2 to every element of the array. But the map function requires a list in the third argument as well. Note: I am putting the add example for simplicity. My original function is much more complicated.
How to use map () to call class methods on a list of objects
Aug 1, 2013 · How to use map for class method within another class method in Python Hot Network Questions Can we slightly modify the Schwarzschild metric to describe an evaporating black hole, and what would the geodesic be like?
Mapping over values in a python dictionary - Stack Overflow
Sep 1, 2012 · The zip function will ensure that all the keys and the values match up correctly while still allowing you to separate the keys from the values so you can apply the map function to only the values. This solution has a time complexity of O(n) with a space complexity of O(1) since we create no new data structures doing this.
python - Map list item to function with arguments - Stack Overflow
Mar 19, 2019 · map will not unpack the args tuple and will pass only one argument (tuple) to myFunc: pool.map(myFunc, args) # map does not unpack the tuple You will need to use multiprocessing.starmap instead. starmap is like map() except that the elements of the iterable are expected to be iterables that are unpacked as arguments. i.e.
python - Use map() function with string - Stack Overflow
May 23, 2018 · Is there a way to use map() function with a string instead of a list? Or the map() function is meant only to work with lists? For instance, ignoring the content of the lambda function, this code r...
Using multiple functions in Python 3 map() - Stack Overflow
map(function, iterable..) However, in place of iterables, you can pass multiple functions and somehow they are treated as iterables. Somehow this line is taking the functions add, square and str and treating them as iterables.