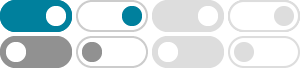
Get a list as input from user in Python - GeeksforGeeks
Dec 5, 2024 · In this article, we will see how to take a list as input from the user using Python. The input() function can be combined with split() to accept multiple elements in a single line and store them in a list. The split() method separates input based on spaces and returns a list. Output:
python - How do I convert user input into a list? - Stack Overflow
user_input = input("Enter String:") magic_list = list(user_input) print(f'magic_list = {magic_list}') This code captures the user input as a string and then converts it into a list of characters using list().
python - Get a list of numbers as input from the user - Stack Overflow
Get a list of number as input from the user. This can be done by using list in python. L=list(map(int,input(),split())) Here L indicates list, map is used to map input with the position, int specifies the datatype of the user input which is in integer datatype, and split() is used to split the number based on space..
Python take a list as input from a user - PYnative
Sep 8, 2023 · Learn how to input a list in Python using input() function. Take list of numbers as well as list strings as input from user
How to Get a List as User Input in Python
Dec 15, 2021 · In this article, we will discuss two ways to get a list as user input in Python. We can get a list of values using the for loop in python. For this, we can first create an empty list and a count variable. After that, we will ask the user for the total count of values in the list.
5 Best Ways to Get a List as Input from User in Python
Mar 11, 2024 · For a Pythonic one-liner approach, you can use a list comprehension combined with input().split() to create a neat single line of code. Here’s an example: number_list = [int(x) for x in input("Enter numbers: ").split()] Assuming a user input of 7 14 21, the output will be: [7, 14, 21] This one-liner uses a list comprehension to split the ...
How to input a list in Python? - Stack Overflow
Apr 8, 2018 · Usually, we input () a list in Python 3.X like this: x = list (map (int, input ())) print (x) But here let's say we give an input of 1234 then it prints:` [1, 2, 3, 4] Is there a way that I can print...
List As Input in Python in Single Line - GeeksforGeeks
Feb 16, 2024 · In Python, there are multiple ways to take a list as input in just a single line of code. Whether you choose list comprehension, map(), direct list(), or a generator expression depends on your preference and specific use case.
How to Input a List in Python using For Loop - GeeksforGeeks
Feb 21, 2025 · Using a for loop to take list input is a simple and common method. It allows users to enter multiple values one by one, storing them in a list. This approach is flexible and works well when the number of inputs is known in advance.
How to Collect List Input from Users in Python
This guide explores various methods for taking list input from users, including techniques for handling strings, numbers, multiple inputs on one line, validating the input and working with multi-dimensional lists.