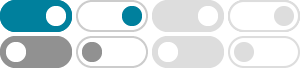
How can I use "e" (Euler's number) and power operation?
Aug 25, 2016 · Python's power operator is ** and Euler's number is math.e, so: from math import e. x.append(1-e**(-value1**2/2*value2**2)) You should use math.exp(stuff) in preference to math.e**stuff. It's likely to be both more accurate and faster. Power is ** and e^ is math.exp: math.e or from math import e (= 2.718281…)
Python math.exp() Method - W3Schools
The math.exp() method returns E raised to the power of x (E x). 'E' is the base of the natural system of logarithms (approximately 2.718282) and x is the number passed to it.
Python math.exp(): Calculate Exponential Values - PyTutorial
Dec 29, 2024 · The math.exp() function in Python's math module calculates the exponential value of a number, specifically e raised to the power of x (e^x), where e is Euler's number (approximately 2.718281828459045).
Python pow() Function - W3Schools
The pow() function returns the value of x to the power of y (x y). If a third parameter is present, it returns x to the power of y, modulus z.
How to calculate e^x in Python - CodeSpeedy
Python offers function pow(base,exponent) to calculate power of number. In this case, pow(base,exponent) function is used calculate x to the power of i.fact(i) computes the factorial of a number. def fact(i): if i==1: return 1 else: return i*fact(i-1)
Creating a exponential function in python - Stack Overflow
Sep 23, 2012 · I want to write a function that takes a single floating-point parameter x and returns the value of the function e (to the power of x) . Using the Taylor series expansion to compute the return value, using a loop that terminates when the partial sum SN+1 of Eq. (2) is equal to SN.
Power and logarithmic functions in Python (exp, log, log10, log2)
Aug 10, 2023 · math.exp(x) returns e to the power of x. math.exp(x) provides a more accurate value than using math.e ** x. To calculate logarithmic functions, use the math.log(), math.log10(), and math.log2() functions. math.log(x, y) returns the logarithm of x with y as the base.
Using Euler's Number & Power Operation in Python - AskPython
Feb 27, 2023 · Enter the exp ( ) function which raises the Euler’s number to the power of the value given as the input. The subtle difference can be found towards the difference in the values of the ending decimals due to the approximation involved in executing this function.
Exponents in Python: A Comprehensive Guide for Beginners
Nov 25, 2024 · The math.exp(x) function computes the exponential value of x, which is equivalent to raising Euler's number e to the power of x. Euler's number is approximately equal to 2.71828, and in mathematical notation, this operation is represented as e^x .
Python Number Exponential Function - Online Tutorials Library
The Python math.exp() method is used to compute the Euler's number 'e' raised to the power of a numeric value. The Eulers number is simply defined as a mathematical expression that is usually used as the base of natural logarithms.