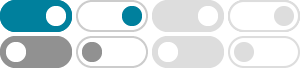
How to format date string via multiple formats in python
May 18, 2014 · Pure python: from datetime import datetime my_datetime = datetime.strptime('2014-05-18', '%Y-%m-%d') repr(my_datetime) >>> 'datetime.datetime(2014,5,18,0,0)' Check datetime.strptime() format …
python - How to change the date format in a list of dates - Stack Overflow
Nov 23, 2015 · You can use a list comprehension together with strftime. datelist = [d.strftime('%Y%m%d') for d in pd.bdate_range(end=pd.datetime.today(), periods = 100)] >>> datelist ['20151110', '20151111', '20151112', '20151113', '20151116', '20151117', '20151118', '20151119', '20151120', '20151123', ...]
How to format multiple date formats into single date in python
Apr 30, 1994 · I have a list in Python with different date formats: list1 = ["30-4-1994", "1994-30-04", "30/04/1994", "30-apr-1994", "30/apr/1994","1994-30-apr"] I want to format multiple date formats into a single date like dd-mm-yyyy. How can I do that?
Formatting Dates in Python - GeeksforGeeks
Mar 15, 2024 · To do this, Python provides a method called strptime(). Syntax: datetime.strptime(string, format) Parameters: string – The input string. format – This is of string type. i.e. the directives can be embedded in the format string. Example: [GFGTABS] Python3
Formatting Date Strings with Multiple Formats in Python 3
Jun 10, 2024 · In this article, we will explore how to handle date strings with multiple formats in Python 3. The datetime.strptime() method in Python’s datetime module allows you to parse date strings based on a specified format.
Python Date Formats: A Comprehensive Guide | by Neeraj …
Jan 16, 2025 · You can mix and match these format codes to create custom date formats. For example: from datetime import datetime now = datetime.now() print(now.strftime("%A, %d %B %Y")) # Output:...
5 Best Ways to Reformat Dates to YYYY-MM-DD Format Using Python
Mar 6, 2024 · This approach utilizes Python’s built-in datetime library, making it both reliable and straightforward to understand. Here’s an example: from datetime import datetime def reformat_date(date_str): date_obj = datetime.strptime(date_str, '%B %d, %Y') return date_obj.strftime('%Y-%m-%d') print(reformat_date('July 4, 2021')) Output: '2021-07-04 ...
5 Best Ways to Display Various Datetime Formats in Python
Mar 7, 2024 · Here we’ll focus on the function strftime(), which allows you to format datetime objects into readable strings. Here’s an example: Output: This code snippet imports the datetime module and retrieves the current local date and time using datetime.now().
Mastering Date & Time Formatting in Python: A Comprehensive …
In this guide, we’ll explore strftime codes, show examples for creating custom date and time formats, and provide tips for working with time zones and localization. The datetime module in Python is the primary tool for handling dates and times. With datetime, you can format dates precisely to match the needs of your application.
Converting a list of dates to a proper date type format in python
Jan 20, 2017 · from datetime import datetime datetime.strptime('Jan2016', '%b%Y') For your list, you can combine the above with a list comprehension: >>> res = [datetime.strptime(d, '%b%Y') for d in dates] >>> [datetime.strftime(d, "%Y%m") for d in res] ['201601', '201602', '201603']