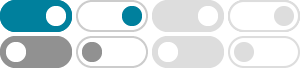
The Fastest Way to Split a Text File Using Python
Jun 5, 2023 · In this post, we’re going to look at the fastest way to read and split a text file using Python. Splitting the data will convert the text to a list, making it easier to work with. We’ll also cover some other methods for splitting text files in Python, and explain how and when these methods are useful.
python - splitting a string based on tab in the file - Stack Overflow
line = "abc def ghi" values = line.split("\t") It works fine as long as there is only one tab between each value. But if there is one than one tab then it copies the tab to values as well.
Python String split() Method - W3Schools
The split() method splits a string into a list. You can specify the separator, default separator is any whitespace.
How to split a text file to its words in python? - Stack Overflow
Mar 11, 1972 · Your answers help me to write this (in my file, words are split by space so that's delimiter I think!): with open ("C:\\...\\...\\...\\record-13.txt") as f: lines = f.readlines() for line in lines: words = line.split() for word in words: print (word)
How to Split a File into a List in Python - GeeksforGeeks
Jun 22, 2022 · In this article, we are going to see how to Split a File into a List in Python. When we want each line of the file to be listed at consecutive positions where each line becomes an element in the file, the splitlines() or rstrip() method is used to split a file into a list.
Reading a text file and splitting it into single words in python
Jun 4, 2013 · Or, if you want a nested list of the words in each line of the file (for example, to create a matrix of rows and columns from a file): with open('words.txt') as f: matrix=[line.split() for line in f] >>> matrix [['line1', 'word1', 'word2'], ['line2', 'word3', 'word4'], ['line3', 'word5', 'word6']]
Read File and Split The Result in Python - PyTutorial
Jun 2, 2023 · In this tutorial, we're going to learn two things: Read a file and split the output. Read a file and split line by line. Before writing our program, let's see the file that we'll read and split. Now let's write our program that reads file.txt and splits the output. txt = f.read() . …
Python Split String: Best Methods and Examples - index.dev
Oct 7, 2024 · Learn effective techniques to split strings in Python, including handling multiple delimiters, splitting by line breaks, and advanced splitting with regular expressions. This guide provides valuable tips and tricks for efficient string manipulation.
Read a text file into a string variable and strip newlines in Python
Aug 1, 2023 · Python Read file into String using split function. The task could also be performed using the split function, a default function in all Python distributions. The function takes in an argument (optional) a character (or string) and splits the …
Fastest Way to Split a Text File Using Python - Online Tutorials …
Feb 1, 2023 · One of the most straightforward ways to split a text file is by using the built-in split () function in Python. Based on a specified delimiter this function splits a string into a list of substrings. For example, the following code splits a text file by newline characters and returns a list of lines ? lines = f.read().split('\n') Here,