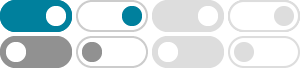
Python Operators Cheat Sheet - LearnPython.com
May 27, 2024 · In this cheat sheet, we will cover every one of Python’s operators: Arithmetic operators. Assignment operators. Comparison operators. Logical operators. Identity operators. Membership operators. Bitwise operators. Additionally, we will discuss operator precedence and its significance in Python.
Python Operators Cheat Sheet Assignment = Assignment a=2 value of a becomes 2 += Addition and assignment i+=1 is the same as i=i+1 -= Subtraction and assignment i-=1 is the same as i=i-1 *= /= etc all other operators can be using in conjunction with the assignment operator Arithmetic operators Operator Description + Addition
Python Basics - Math Cheat Sheet - Cheatography.com
# This script solves ax^2 + bx + c = 0 import math a = 1 b = -1 c = -6 delta = b*2 - 4a*c r1 = (-b + math.sqrt(delta))/(2*a) r2 = (-b - math.sqrt(delta))/(2*a) print(f"r1 = {r1}") print(f"r2 = {r2}") r1 = 3.0
Python Cheat Sheet & Quick Reference
The Python cheat sheet is a one-page reference sheet for the Python 3 programming language.
angle2 = math.a cos (si de2 /side3) print( f"angle 1 = {math.d eg ree s(a ngl e1) }") print( f"angle 2 = {math.d eg ree s(a ngl e2) }")
logic operations (True/False). Math Operations In Python In python math operations are very similar to your cal-culator. Below is a table summarizing basic operations through illustrated example: Operation Mathematics Python Addition a+ b a+b Subtraction a+ b a-b Multiplication a b a*b Division a b a=b Exponentiation ab a**b
- [PDF]
Python Cheat Sheet
Python Cheat Sheet The print() Function The print() function prints the specified message to the screen, or other output device. The message can be a string, or any other object that is converted into a string before being written to the screen. print("Hello World!") Printing a math solution x = 9 y = 2 print("Sum: " + str(x + 9))
for operations f.write("coucou") f.writelines(list of lines) writing reading f.read([n]) → next chars if n not specified, read up to end ! f.readlines([n]) → list of next lines f.readline() → next line with open(…) as f: for line in f : # processing ofline cf. modules os, os.path and pathlib f.close() ☝ dont forget to close the file ...
Python Cheat Sheet - The Ultimate Guide - vivitoa.github.io
Learn Python quickly with this complete cheat sheet covering variables, loops, functions, OOP, web scraping, and more.
Download Python Cheat Sheet PDF for Quick Reference
Click here to download the Python Cheat Sheet PDF. 1. Math Operators. You can perform math operations like addition, subtraction, multiplication, and division using arithmetic operators in Python. You can also access several libraries that can …
- Some results have been removed