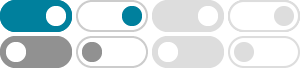
Python Socket Programming: Server and Client Example Guide
Feb 21, 2025 · In this tutorial, you will learn the basics of Python socket programming, including how to create a simple client-server architecture, handle multiple clients using threading, and understand the differences between TCP and UDP sockets.
Basic Python client socket example - Stack Overflow
Here is the simplest python socket example. Server side: connection, address = serversocket.accept() buf = connection.recv(64) if len(buf) > 0: print buf. break. Client Side: How would I do this cross-computer on a LAN connection? I'm having trouble.
Socket Programming in Python - GeeksforGeeks
Feb 28, 2023 · The server forms the listener socket while the client reaches out to the server. They are the real backbones behind web browsing. In simpler terms, there is a server and a client. Socket programming is started by importing the socket library and making a simple socket. import socket s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
Socket Programming in Python (Guide) – Real Python
Dec 7, 2024 · In this in-depth tutorial, you'll learn how to build a socket server and client with Python. By the end of this tutorial, you'll understand how to use the main functions and methods in Python's socket module to write your own networked client-server applications.
Socket Programming HOWTO — Python 3.13.3 documentation
1 day ago · So first, let’s make a distinction between a “client” socket - an endpoint of a conversation, and a “server” socket, which is more like a switchboard operator. The client application (your browser, for example) uses “client” sockets exclusively; the web server it’s talking to uses both “server” sockets and “client” sockets.
Python simple socket client/server using asyncio
I would like to re-implement my code using asyncio coroutines instead of multi-threading. server.py. request = None. while request != 'quit': request = client.recv(255).decode('utf8') response = cmd.run(request) client.send(response.encode('utf8')) client.close() while True: client, _ = server.accept()
A Complete Guide to Socket Programming in Python
Aug 18, 2023 · In this article, we will cover the basics of socket programming and provide a step-by-step guide to creating socket-based client and server applications using Python. So without further ado, let's dive right in! Networking enables communication and information sharing of …
Guide To Socket Programming in Python: Easy Examples
With the basics out of the way, you're ready to implement a simple client and server. The server will echo (send back) the received data to the client. Let's begin with the server implementation: s.bind((HOST, PORT)) s.listen() conn, addr = s.accept() with conn: print(f"Connected by {addr}")
Building on Python Socket Applications: Simple Client-Server
Sep 10, 2024 · In this post, we’ll build a simple TCP socket server that opens a door for clients and responds with a welcome message. We’ll also create a client that knocks on that door and receives the...
An Essential Guide to Socket Programming in Python: Client, Server…
Mar 6, 2023 · In this tutorial, you'll learn how to exchange data between a client and a server using Python socket programming and the Socket API. Later, this tutorial will discuss exchanging data directly between two or more Python clients using a hosted provider.
- Some results have been removed