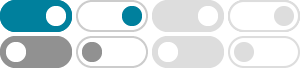
Reversing a String with Recursion in Java - Stack Overflow
public static String reverse(String str) { return (str == null || str.length()==0) ? str : reverseString2(str.substring(1))+str.charAt(0); }
Print reverse of a string using recursion - GeeksforGeeks
Mar 6, 2025 · Given a string, the task is to print the given string in reverse order using recursion. Examples: Input: s = “Geeks for Geeks” Output: “ skeeG rof skeeG “ Explanation: After reversing the input string we get “ skeeG rof skeeG “. Input: s = “Reverse a string Using Recursion” Output: “ noisruceR gnisU gnirts a esreveR “
Whats the best way to recursively reverse a string in Java?
May 14, 2009 · String reverse(String str) { if(str.length()<2) return str; return reverse(str.substring(1)) +str.charAt(0); } and tail recursive version: String reverseTail(String str) { if(str.length()<2) return str; return str.charAt(str.length()-1)+ …
Java Program to Reverse a String Using Recursion
Mar 11, 2021 · In this program, we will see how to reverse a string using recursion with a user-defined string. Here, we will ask the user to enter the string and then we will reverse that string by calling a function recursively and finally will print the reversed string. Declare a string. Ask the user to initialize the string.
Java Program to Reverse a String using Recursion
Sep 8, 2017 · We will see two programs to reverse a string. First program reverses the given string using recursion and the second program reads the string entered by user and then reverses it. To understand these programs you should have the knowledge of following core java concepts: 1) substring() in java 2) charAt() method Example 1: Program
Reverse a String Using Recursion in Java - Tpoint Tech
Mar 26, 2025 · In this section, we will learn how to reverse a string using recursion in Java. First, remove the first character from the string and append that character at the end of the string. Repeat the above step until the input string becomes empty. Suppose, the input string JAVATPOINT is to be reversed.
How to Reverse a String in Java using Recursion - Guru99
Dec 30, 2023 · In this program, we will reverse a string entered by a user. We will create a function to reverse a string. Later we will call it recursively until all characters are reversed.
How to Reverse a String Recursively in Java: Detailed Explanation
Reversing a string using recursion in Java involves breaking down the string and recursively processing its characters from the back to the front. In this method, the string is reduced in size with each recursive call until it reaches the base case of a single character or an empty string.
How to Reverse a String in Java using Recursion: An Expert‘s Guide
Jan 27, 2025 · Here is the full Java code snippet to recursively reverse a string: public static String reverse(String input) { // Base case. if (input.equals("")) { return ""; // Recursive case . else { // Extract first char . char first = input.charAt(0); . // Reverse substring after first char recursively.
Reverse All Characters of a String in Java - HowToDoInJava
Jan 10, 2023 · Learn to reverse all the characters of a Java string using the recursion and StringBuilder.reverse() methods.
- Some results have been removed