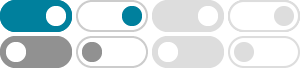
Reverse a number using stack - GeeksforGeeks
Jul 3, 2024 · In this post we will discuss about how to reverse a number using stack. The idea to do this is to extract digits of the number and push the digits on to a stack. Once all of the digits of the number are pushed to the stack, we will start popping the contents of …
Reversing a stack in java
Jun 6, 2017 · If you keep popping elements off the stack until a.empty() returns true, then you have emptied the stack, and you are passing an empty stack to the reverse method. Why not just use: System.out.println(a); reverse(a);
Reversing a Stack in Java - Baeldung
Apr 19, 2024 · In this article, we’ll look into the different ways of reversing a stack using Java. A stack is a LIFO(Last In, First Out) data structure that supports the insertion (push) and removal (pop) of elements from the same side. We can visualise a stack as a stack of plates on a table; accessing the plates is the safest when done from the top. 2.
Java reverse an int value without using array - Stack Overflow
Apr 29, 2017 · Java reverse an int value - Principles. Modding (%) the input int by 10 will extract off the rightmost digit. example: (1234 % 10) = 4. Multiplying an integer by 10 will "push it left" exposing a zero to the right of that number, example: (5 * 10) = 50. Dividing an integer by 10 will remove the rightmost digit. (75 / 10) = 7
Reverse Number Program in Java - GeeksforGeeks
Apr 8, 2025 · We can reverse a number in Java using three main methods as mentioned below: 1. Using While Loop. Simply apply the steps/algorithm discussed and terminate the loop when the number becomes zero. Example: The complexity of the above method: 2. Using Recursion. In recursion, the final reverse value will be stored in the global ‘rev’ variable.
stack - arrayImpOfStack.java how to reverse number in output? - Stack …
Mar 16, 2021 · Reverse the elements in an array and push the reversed elements onto a stack using a single for loop
Reverse Numbers with a Stack – Java Task (Stacks) – Java
Write a program that reads N integers from the console and reverses them using a stack. Use the Stack<Integer> class. Just put the input numbers in the stack and pop them.
Java: Reverse the elements of a stack - w3resource
Mar 11, 2025 · Write a Java program to reverse a stack in place by swapping elements from the top and bottom recursively. Write a Java program to implement an algorithm that reverses a stack using only one additional temporary variable.
Reverse a Number using Stack | Stacks | PrepBytes Blog
Sep 28, 2022 · Reverse a number using stack is a straightforward process that involves extracting each digit of the number and inserting it into the stack one by one. The Last In First Out (LIFO) property of the stack ensures that the digits are reversed in the correct order.
How to Reverse a Stack in Java - Java Guides
In this article, we will write a simple program to reverse a Stack using recursion. we are not using loop constructs like a while, for etc, and we only use the following ADT functions on Stack: Let's write generic methods so that we can reverse any data type like String, Integer, Double etc.