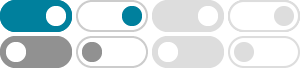
How do I append one string to another in Python?
Dec 14, 2010 · If you only have one reference to a string and you concatenate another string to the end, CPython now special cases this and tries to extend the string in place. The end result is that the operation is amortized O(n). e.g. s = "" for i in range(n): s += str(i) used to be O(n^2), but now it is O(n). More information. From the source (bytesobject.c):
How can I selectively escape percent (%) in Python strings?
May 21, 2012 · Alternatively, as of Python 2.6, you can use new string formatting (described in PEP 3101): 'Print percent % in sentence and not {0}'.format(test) which is especially handy as your strings get more complicated.
Is there a "not equal" operator in Python? - Stack Overflow
Jun 16, 2012 · Python is dynamically, but strongly typed, and other statically typed languages would complain about comparing different types. There's also the else clause: # This will always print either "hi" or "no hi" unless something unforeseen happens. if hi == "hi": # The variable hi is being compared to the string "hi", strings are immutable in Python ...
python - How to change any data type into a string ... - Stack …
Jul 8, 2010 · The rule of thumb for repr is that if it makes sense to return Python code that could be evaluated to produce the same object, do that, just like str, frozenset, and most other builtins do, but if it doesn't, use the angle-bracket form to make sure that what you return can't possibly be mistaken for a human-readable-and-evaluatable-as-source repr.
How do I get a substring of a string in Python? - Stack Overflow
Aug 31, 2016 · @gimel: Actually, [:] on an immutable type doesn't make a copy at all. While mysequence[:] is mostly harmless when mysequence is an immutable type like str, tuple, bytes (Py3) or unicode (Py2), a = b[:] is equivalent to a = b, it just wastes a little time dispatching the slicing byte codes which the object responds to by returning itself since it's pointless to shallow copy when, aside from ...
String formatting in Python - Stack Overflow
Use Python 3 string formatting. This is still available in earlier versions (from 2.6), but is the 'new' way of doing it in Py 3. Note you can either use positional (ordinal) arguments, or named arguments (for the heck of it I've put them in reverse order.
How to get the size (length) of a string in Python
Aug 31, 2023 · Python 2: It gets complicated for Python 2. A. The len() function in Python 2 returns count of bytes allocated to store encoded characters in a str object. Sometimes it will be equal to character count: >>> print(len('abc')) 3 But sometimes, it won't: >>> print(len('йцы')) # String contains Cyrillic symbols 6
Convert integer to string in Python - Stack Overflow
Jun 23, 2019 · For Python 3.6, you can use the f-strings new feature to convert to string and it's faster compared to str() function. It is used like this: It is used like this: age = 45 strAge = f'{age}'
python - How to check if type of a variable is string? - Stack …
Jan 30, 2011 · Use type() or isinstance(). I don't know why not a single answer before me contains this simple type(my_variable) is str syntax, but using type() like this seems the most-logical and simple to me, by far:
python - how to find whether a string is contained in another …
that's it as far as all the info i have for now. however, if you are learning Python and/or learning programming, one highly useful exercise i give to my students is to try and build *find() and *index() in Python code yourself, or even in and not in (although as functions). you'll get good practice traversing through strings, and you'll have a ...